Vue Js Image Slide Show: Vue.js is a progressive JavaScript framework used to build user interfaces. With Vue.js, you can easily create an image slideshow by utilizing its reactive data binding, components, and directives. A typical image slideshow in Vue.js involves creating an array of image URLs, and then displaying them in a component using v-for directive. You can also add a computed property to the component to implement a timer to automatically switch images. To add animation to the slideshow, you can use Vue.js transitions. With Vue.js, creating a dynamic and responsive image slideshow is a straightforward process that can enhance user experience on your website or application.
Simple Image Slider Using Vue.js for Web Design
How to create a simple image slider using Vue.js for web design?
this is a Vue.js component that creates a simple slider to display a sequence of images. The images are stored in an array and are displayed one at a time, with the current image index determined by the ‘currentImageIndex’ data property.
To create the slider functionality, the component uses two methods: prevImage() and nextImage(). These methods allow the user to navigate through the images by decrementing or incrementing the ‘currentImageIndex’ respectively.
To enable automatic rotation of the images, the component uses the ‘setInterval()’ method within the ‘created()’ lifecycle hook. This updates the ‘currentImageIndex’ every 5 seconds, effectively rotating the images in a loop.
Vue Js Carousel Example
<div id="app">
<div class="slider">
<img :src="images[currentImageIndex]" alt="Slider Image" class="slider-image">
<div class="controls">
<button class="prev-btn" @click="prevImage">
<i class="fa fa-chevron-left"></i>
</button>
<button class="next-btn" @click="nextImage">
<i class="fa fa-chevron-right"></i>
</button>
</div>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
currentImageIndex: 0,
images: [
'https://www.sarkarinaukriexams.com/images/editor/1670265927Capture123243243423.PNG',
'https://www.sarkarinaukriexams.com/images/post/1670772103thisisengineering-raeng-yhCHx8Mc-Kc-unsplash_(1).jpg',
'https://www.sarkarinaukriexams.com/images/post/1673249449chnange_img_src_dynamically_(1).jpg',
'https://www.sarkarinaukriexams.com/images/editor/1670265927Capture123243243423.PNG',
'https://www.sarkarinaukriexams.com/images/post/1670772103thisisengineering-raeng-yhCHx8Mc-Kc-unsplash_(1).jpg',
'https://www.sarkarinaukriexams.com/images/editor/1670265927Capture123243243423.PNG'
],
};
},
created() {
setInterval(() => {
this.currentImageIndex = (this.currentImageIndex + 1) % this.images.length;
}, 5000);
},
methods: {
prevImage() {
this.currentImageIndex = (this.currentImageIndex === 0) ? this.images.length - 1 : this.currentImageIndex - 1;
},
nextImage() {
this.currentImageIndex = (this.currentImageIndex === this.images.length - 1) ? 0 : this.currentImageIndex + 1;
}
},
});
app.mount('#app');
</script>
Output of Vue Js Image slide show
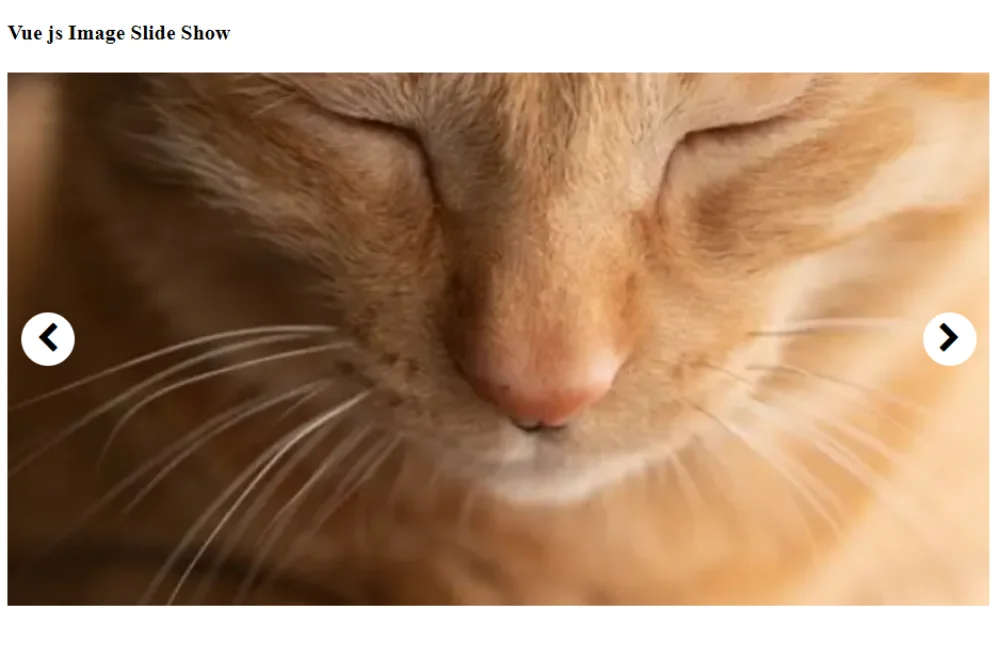